Geujes CG Staff
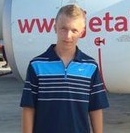
Posts : 34 Join date : 2009-09-26 Age : 29 Location : Belgium
 | Subject: C++ Super Basics Sat Sep 26, 2009 7:17 pm | |
| CrazyGamerz Gunz,C++ Super Basics !If you're serious, here's a free C++ compiler that actually kicks ass: Bloodshed Dev C++, http://bloodshed-dev-c.en.softonic.com/Start with: - Code:
-
#include <iostream> using namespace std; int main() {
} NOTE: #include is the same as import in Java. NOTE: std doesn't stand for what you think. Add a pause to the program. - Code:
-
system("pause"); Add a variable. possible data types - int - bool - long - string - char - float - Code:
-
int variblename; bool variablename; long variablename; string variablename; char variablename; float variablename; comments: - Code:
-
/* COMMENTS FTW */ Assigning values to variables: - Code:
-
int charlie = 1; COnditional statements: - Code:
-
int a = 1; int b = 1; if (a == b) { /* "==" is the comparison operator and is necessary here */ } while (a == b) {
}
/* else */
if (a == b) {
} else {
}
/* else if */
if (a == b) {
} else if (a != b) /* "!=" is not equal to */ {
}
/* and's and or's, and = &&, or = || */
if (a == b && b == c) { a = c; } input and output. - Code:
-
/* cout = consule out */ /* that outputs text */
cout<<"Hello, World!";
/* you can also add a line break. "endl" means end line */
cout<<"Hello, World!"<<endl;
/* cin = consule in */ /* that inputs text so that the user assigns the value during the program */
int x; cin>>x; cout<<x; /* displays the number you just inputed. */ Math operators - Code:
-
int x = 2; int y = 3; int z; z = x + y; cout<<z; /* since x + y means 2 + 3, then z will become 5. */ /* then the program displays 5 */ /* Addition: + Subtraction: - Multiplication: * Division: / */ Functions writing a separate function allows you do carry out a task of code outside your main set of code to keep it lookin smexie. - Code:
-
int addition(int x, int y) /* this is the actual function */ { int z; z = x + y; return z; }
int main() { int x = 2; int y = 3; int z = addition(x, y); /* This is a called function */ cout<<z; } As you can see, we call the function in the main and we put x and y in it's ()'s. This allows the above function to absorb the sexiness of the called funcion and use it for itself. Then it returns the sexiness back to the called function so that the main can have it's way with it. Clear the consule screen and start fresh. - Code:
-
system("cls"); /* cls = clear screen */ Pwning Spongebob. system("spongebob/pwn"); ^that was a joke. It's not actually part of C++. Structures. Creating structures is like making a main category of something. and then you assign all the subcategories. For example, K-Style is a main category and BF, SS, HS are subcategories. - Code:
-
struct Kstyle { int BF; int SS; int HS; }
int main() { /* Notice the name of the structure is Kstyle. That becomes what looks like the "datatype". Then make up whatever name you want for the higher class. */ Kstyle Moves; Moves.BF = 1; Moves.SS = 2; Moves.HS = 3;
double x = Moves.BF + (Moves.SS * Moves.HS); cout<<x; system("pause"); } CrazyGamerz Team ! | |
|